When you declare a variable in C# it will be saved as either a Value or Reference Type.
These two types refer to how the variable is stored in memory on the computer.
Value Types
Value types store the value within it's own memory space, that is to say that the memory location that the variable is pointing to contains the actual value.
Let's take a look at an example.
We'll declare an integer i with a value of 100. The computer will then store the value 100 in the memory location that the variable i is pointing to:
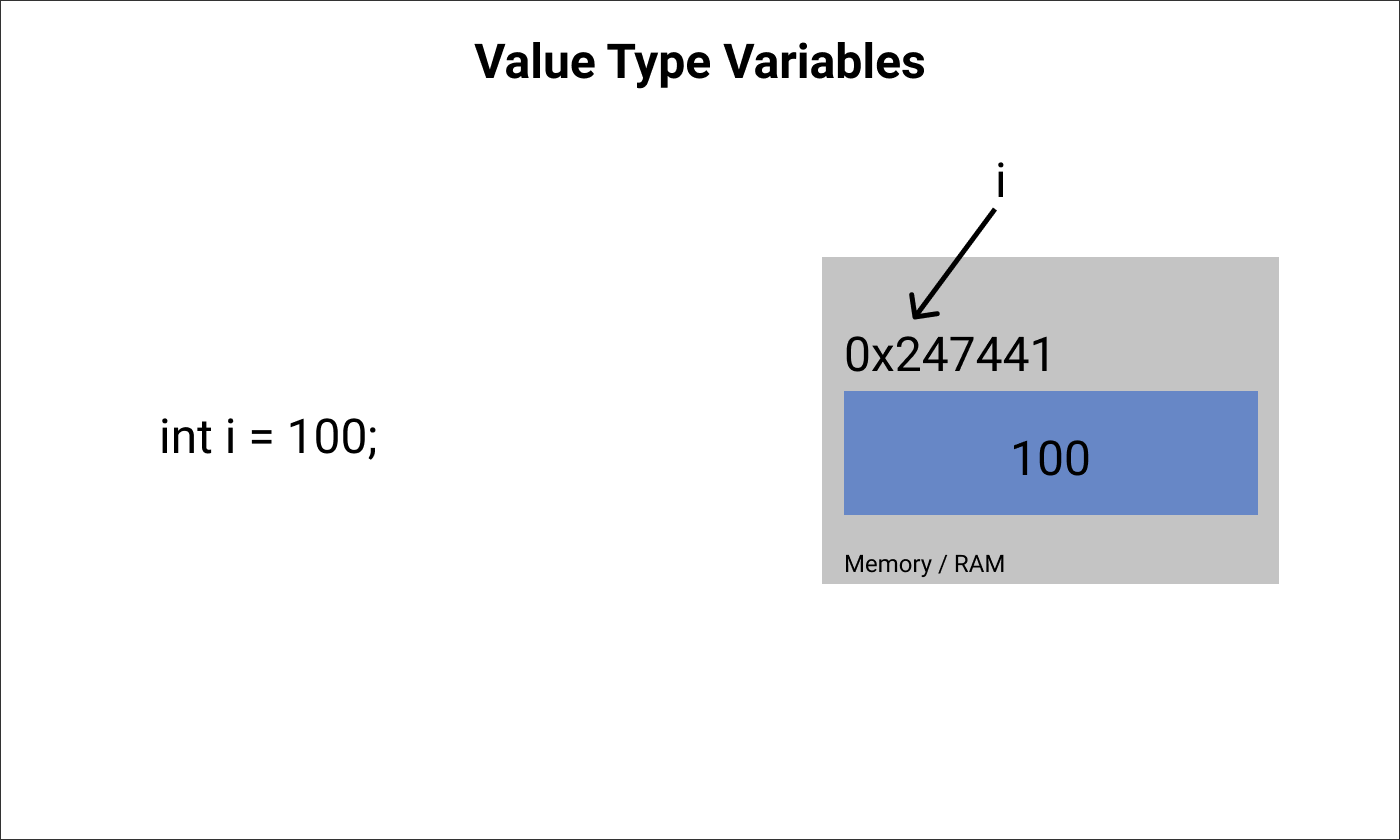
Reference Types
Reference types store their values in a different way to value types. Reference types store the address of where the value is being stored.
Let's demonstrate with another example.
Declare a string s = "Hi". The computer will store s in a location; 0x247439 in our case. The value of s is 0x247442 which is just the address of the actual data value.
s is just a reference to where the actual data is stored.
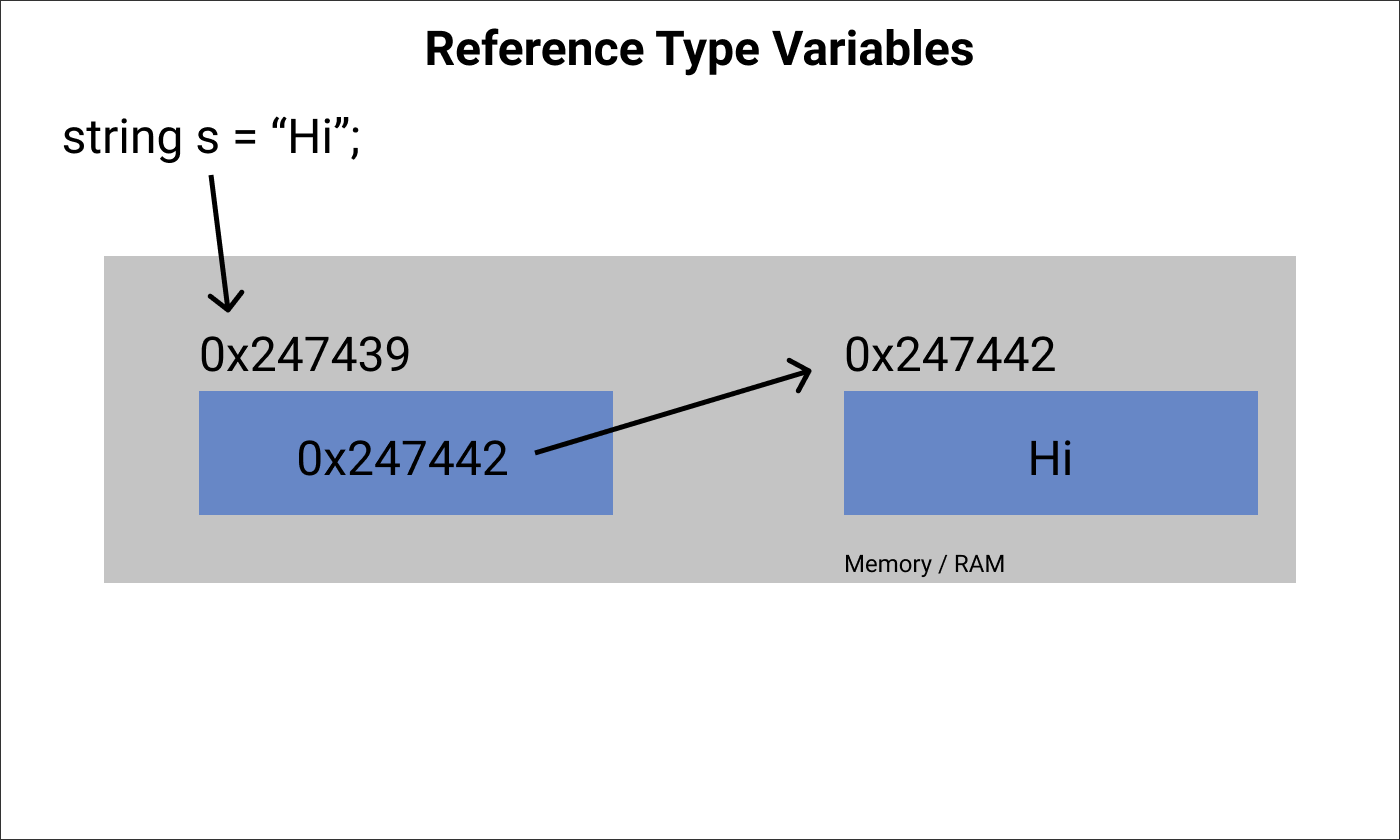
Simple Reference Type Test
Here's a simple test that shows that two variables can reference the same object:
[Fact]
public void TwoVarsCanReferenceSameObject()
{
// arrange
var book1 = GetBook("Book 1");
var book2 = book1;
// assert
Assert.Same(book1, book2);
Assert.True(Object.ReferenceEquals(book1, book2));
}
InMemoryBook GetBook(string name)
{
return new InMemoryBook(name);
}
Built-In Types for the C# Language
C# type keyword | .NET type |
---|---|
bool | System.Boolean |
byte | System.Byte |
sbyte | System.SByte |
char | System.Char |
decimal | System.Decimal |
double | System.Double |
float | System.Single |
int | System.Int32 |
uint | System.UInt32 |
nint | System.IntPtr |
nuint | System.UIntPtr |
long | System.Int6 |
ulong | System.UInt64 |
short | System.Int16 |
ushort | System.UInt16 |
The following table lists the C# built-in reference types:
C# type keyword | .NET type |
---|---|
object | System.Object |
string | system.String |
dynamic | System.Object |
The preceding two tables were taken from https://learn.microsoft.com/en-us/dotnet/csharp/language-reference/builtin-types/built-in-types
Special Case:
String is defined by a class in C#. It is always a Reference type, but it behaves like a value type.
Strings are immutable. This is a key point!
Chat soon.
Comments