Let's clear up any confusion you have related to the LINQ methods Any(), All() and Contains().
They're extremely useful for querying (asking questions about) your data. I'll first explain how they work at a high level and then give an example of each one.
Important: All three of these methods return a boolean (true/false). Your result will either be true or false.
The table of Countries will be used to demonstrate how these LINQ Methods can be used. We'll say the name of the collection is 'countries'.
countries
Id | Name | Capital | Population | Language | HasMaleLeader |
---|---|---|---|---|---|
1 | Ireland | Dublin | 4,900,000 | English | True |
2 | USA | Washington, D.C. | 309,000,000 | English | True |
3 | Sweden | Stockholm | 9,000,000 | Swedish | True |
4 | New Zealand | Wellington | 4,900,000 | English | False |
5 | France | Paris | 67,000,000 | French | True |
LINQ Any()
"Tell me if any of the things in this collection match this condition"
Id | Name | Capital | Population | Language | HasMaleLeader |
---|---|---|---|---|---|
1 | Ireland | Dublin | 4,900,000 | English | True |
2 | USA | Washington, D.C. | 309,000,000 | English | True |
3 | Sweden | Stockholm | 9,000,000 | Swedish | True |
4 | New Zealand | Wellington | 4,900,000 | English | False |
5 | France | Paris | 67,000,000 | French | True |
For example, are there any countries in this list that have a population over 300,000,000?
var countryExistsWithPopulationOver300 = countries.Any(country => country.Population > 300000000);
Console.WriteLine(countryExistsWithPopulationOver300);
"True"
So in the above example we're setting the value of countryExistsWithPopulationOver500 to the result of the LINQ query.
Since the USA has a population of over 300,000,000 the value for countryExistsWithPopulationOver300 would be set to true.
The Any() method can also be used to quickly check if a collection has elements within it.
var listIsNotEmpty = countries.Any();
Console.WriteLine(listIsNotEmpty);
"True"
listIsNotEmpty would be true as the countries.Any() would be return true.
Extra information regarding Any: the Any() method is lazy meaning that as soon as one element or "thing" (country in our case) in the collection returns true, it stops iterating through the rest of the collection. This improves performance.
In our example above, Ireland would be the first element to be checked against the condition; result = false. The USA would be the next element to be checked; result = true.
The Any method would then return and would not check Sweden or France against the condition in order to save time.
The default value of Any is false, as soon as it finds an item that is satisfies the predicate (country => country.Population > 300000000), the method stops and returns true.
Here's a quick sketch I made showing how I visualise the method working. You can think of it as some observer (the eyes) checking each item as it passes through the narrow passage. The observer then checks to see if that item matches the condition (Colour is red). If it is true the then observer can report back with the result TRUE.
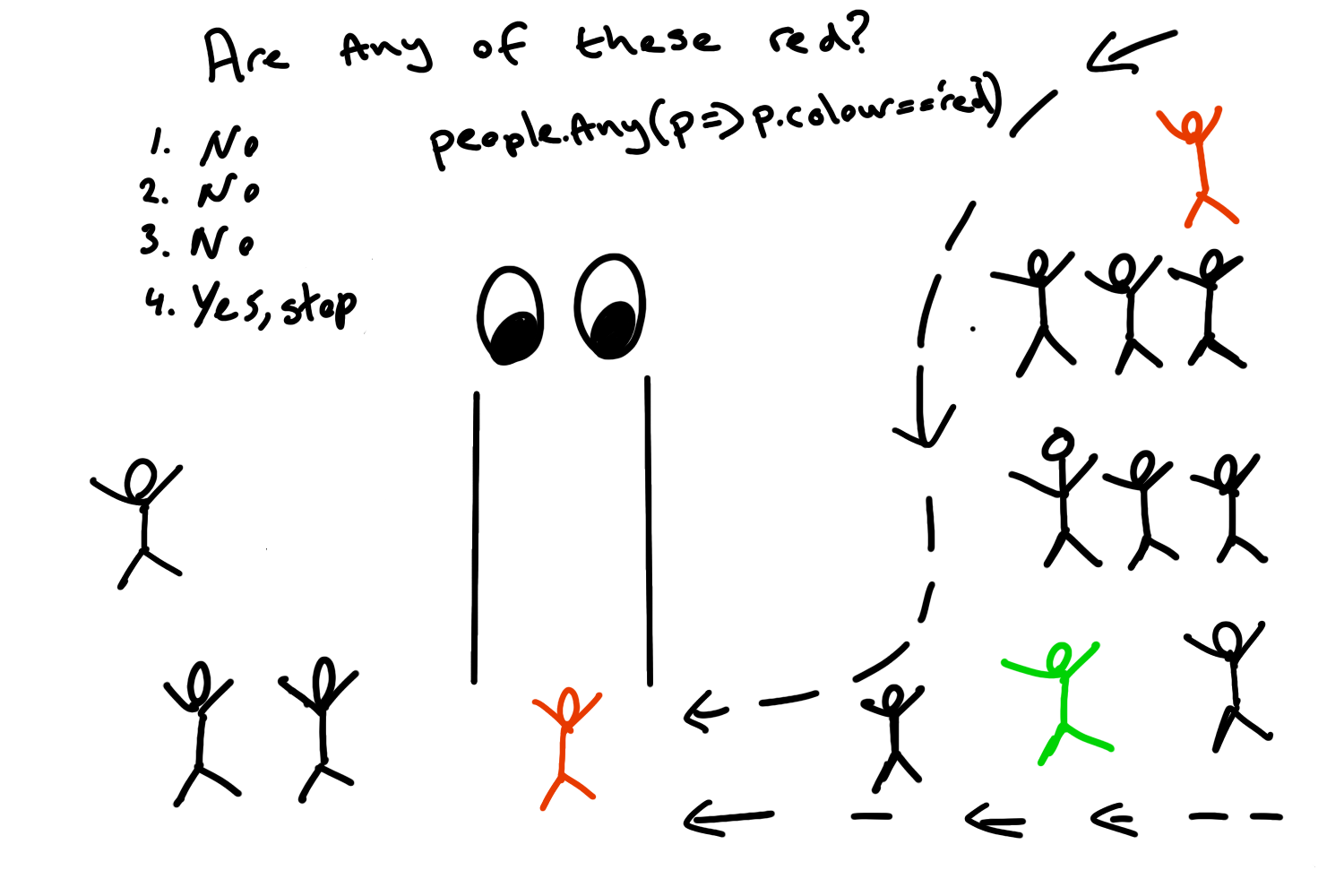
LINQ All()
"Tell me if all of the things in this collection match this condition"
The opposite of Any()? Kind of..
Id | Name | Capital | Population | Language | HasMaleLeader |
---|---|---|---|---|---|
1 | Ireland | Dublin | 4,900,000 | English | True |
2 | USA | Washington, D.C. | 309,000,000 | English | True |
3 | Sweden | Stockholm | 9,000,000 | Swedish | True |
4 | New Zealand | Wellington | 4,900,000 | English | False |
5 | France | Paris | 67,000,000 | French | True |
Example, do all of the countries in the list have a male leader?
var allCountriesHaveMaleLeader = countries.All(country => country.HasMaleLeader == true);
Console.WriteLine(allCountriesHaveMaleLeader);
"False"
Since New Zealand has a female leader the value for allCountriesHaveMaleLeader would be set to false.
Like the Any() method, the All() method is lazy. The default value for All() is true, as soon as it finds an element in the collection that fails it will stop and return false.
In our countries example, Any() would have checked Ireland, USA, Sweden and New Zealand. Since the result for New Zealand was false, it would stop there and return true. It would not check France.
LINQ Contains()
"Tell me if this object is in this collection"
Contains is similar to Any(). However, Contains() can only accept objects whereas Any() is that bit more flexible.
There is an overload for Contains() but I won't go into that here.
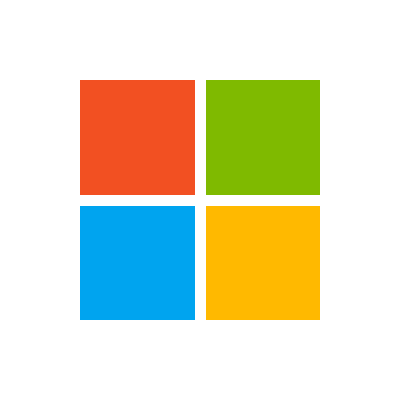
Let's demonstrate this.
Id | Name | Capital | Population | Language | HasMaleLeader |
---|---|---|---|---|---|
1 | Ireland | Dublin | 4,900,000 | English | True |
2 | USA | Washington, D.C. | 309,000,000 | English | True |
3 | Sweden | Stockholm | 9,000,000 | Swedish | True |
4 | New Zealand | Wellington | 4,900,000 | English | False |
5 | France | Paris | 67,000,000 | French | True |
First we'll get a reference to a Country object, then we'll use the Contains() method to check to see if it exists in the collection.
This example is trivial as we're retrieving the sweden object from the list in the first place so we know it's there.
var sweden = countries.First(country => country.name == 'Sweden');
var isSwedenInCollection = countries.Contains(sweden);
Console.WriteLine(isSwedenInCollection);
"True"
Since the sweden object is in the collection, the result is true.
Like the other two methods, Contains() is lazy. The default value is false, as soon as the predicate is satisfied (it finds sweden), it stops and returns true.
That's it for my summary on the three LINQ methods Any(), All() and Contains(). I hope it was of some use to you. Let me know in the comments if it helped out or if I've missed something you think should be included.
You can follow me on twitter @eamokeane where I'll continue to document my journey as a software developer.
Comments